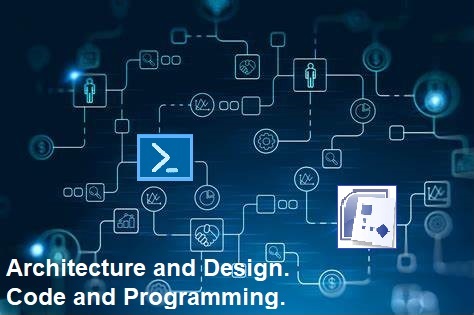
Introduction
Hello to all readers of the blog about IT Architecture and Education.
We continue publishing articles under the conceptual title “Architecture and Design. Implementation and Operation. Code and Programming”.
In the last article, it was about displaying the Microsoft System Center Virtual Machine Manager 2007 system architecture diagram in Visio format for the corresponding series of articles. We did this work using Powershell code. The result fully met our expectations. However, the main significant drawback is the amount of code and the lack of a strategy for its reuse. With the help of functions, you can organize blocks of code for reuse and reduce its overall volume.
This post will be dedicated to this.
Powershell functions
The implementation of Powershell functions requires the placement of this code in a file and the corresponding command syntax and initialization of these elements. So, let’s create a new file PSVisio.v0.1.ps1 in which all our work will be placed.
In order to initialize the code of the Powershell functions written by us, the following command should be executed:
. .\PSVisio.v0.1.ps1
When making changes to the code, the modified function should be unloaded by the command:
rm Function:\NameOfFunction
And perform the initialization again with the command described above.
At the beginning of our code writing and function filling, the PSVisio.v0.1.ps1 file will contain a header in the form of comments and the initial initialization of several variables. They will be used in the formation of the name of the object by consecutive numbering:
#######################################################################
#
# Powershell functions for operate Visio Drawing
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 14.09.2007
# Purpose/Change: Initial script development.
# Create function New-VisioApplication.
#
# Run:
#
# . .\PSVisio.v0.1.ps1
#
#######################################################################
# Set Variables
$Shape=0
$Line=0
$Icon=0
$Text=0
$PolyLine=0
New-VisioApplication
Let’s recall the initial piece of code that we described in the last post:
# Step 1.
# Create Visio Object
# Create Document from Blank Template
# Set Active Page
$Application = New-Object -ComObject Visio.Application
$Application.Visible = $True
$Documents = $Application.Documents
$Document=$Application.Documents.Add('')
$Page=$Application.ActivePage
$Application.ActivePage.PageSheet
# Step 2.
# Add Basic Visio Stensils
# Set Masters Item Rectangle
$Stensil1 = $Application.Documents.Add("BASIC_M.vss")
$Rectangle = $Stensil1.Masters.Item("Rectangle")
As you can see, it starts with the operators for creating the Visio Application Object. Let’s formalize these simple steps in the form of the first function – New-VisioApplication.
Function New-VisioApplication {
#######################################################################
#
# Powershell function for create Visio Application
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 14.09.2007
# Purpose/Change: Initial script development
#
# Run:
#
# New-VisioApplication
#
#######################################################################
# Create Visio Object
$Script:Application = New-Object -ComObject Visio.Application
$Script:Application.Visible = $True
}
The function turned out to be quite simple and does not involve working with any parameters. Do not forget to make comments with explanations to your code.
New-VisioDocument
We will describe the following function for creating a new Visio document: New-VisioDocument.
The function will create a new Visio Document based on an empty template. This function also has no input parameters.
Function New-VisioDocument {
#######################################################################
#
# Powershell function for create Visio Document
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 14.09.2007
# Purpose/Change: Initial script development
#
# Run:
#
# New-VisioDocument
#
#######################################################################
# Create Document from Blank Template
$Script:Documents = $Script:Application.Documents
$Script:Document=$Script:Application.Documents.Add('')
}
Set-VisioPage
The Set-VisioPage function is also straightforward. Its logic is in setting the Active page, which will be the basis of our future scheme. The function also has no input parameters.
Function Set-VisioPage {
#######################################################################
#
# Powershell function for Set Active Visio Document Page
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 14.09.2007
# Purpose/Change: Initial script development
#
# Run:
#
# Set-VisioPage
#
#######################################################################
# Set Visio Active Page
$Script:Page=$Script:Application.ActivePage
$Script:Application.ActivePage.PageSheet
}
Add-VisioStensil
In Visio, shapes are based on stencils. Therefore, the task of the following Add-VisioStensil function is to add shape stencils from the corresponding .vss file. The function receives two input parameters: Name – the name for the stencil object, and File – the name of the stencil file.
Function Add-VisioStensil ($Name, $File) {
#######################################################################
#
# Powershell function for Add Visio Stensil
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 15.09.2007
# Purpose/Change: Initial script development
#
# Run:
#
# Add-VisioStensil -Name "Basic" -File "BASIC_M.vss"
#
#######################################################################
# Set Expression and Add Visio Stensil
$Expression = '$Script:' + $Name + ' = $Script:Application.Documents.Add("' + $File +'")'
Invoke-Expression $Expression
}
Set-VisioStensilMasterItem
To work with a specific figure, you should specify what will be the main sample of the figure. We will design the logic of this operation in the form of the Set-VisioStensilMasterItem function. The function has two input parameters: Stensil – the name of the stencil object and Item – the name of the figure.
Function Set-VisioStensilMasterItem ($Stensil, $Item) {
#######################################################################
#
# Powershell function for Set Stensil Master Item
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 15.09.2007
# Purpose/Change: Initial script development
#
# Version: 0.2
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 17.09.2007
# Purpose/Change: Reorganize Variables
#
# Run:
#
# Set-VisioStensilMasterItem -Stensil "Basic" -Item "Rectangle"
#
#######################################################################
# Set Expression And Set Masters Item Rectangle
$ItemWithoutSpace = $Item -replace " ",""
$Expression = '$Script:' + $ItemWithoutSpace + ' = $Script:' + $Stensil + '.Masters.Item("' + $Item + '")'
Invoke-Expression $Expression
}
Draw-VisioItem
So we came directly to the functions that will perform the actions of creating and displaying objects with the specified characteristics. To begin with, these will be simple geometric shapes – rectangle, circle, line, etc.
Let’s recall again the code fragment that performs these actions.
# Step 3.
# Draw Main Rectangle, Set Size, Set Colour
# Set Text, Size, Color, Align
# Draw Line, Set Weight, Color
$Shape1 = $Page.Drop($Rectangle, 6.375, 7.125)
$Shape1.Cells('Width').Formula = '12.2501'
$Shape1.Cells('Height').Formula = '7.25'
$Shape1.Cells('FillForegnd').Formula = '=RGB(0,153,204)'
$Shape1.Cells('LinePattern').Formula = 0
$Shape1.Text = "Microsoft Virtual Machine Manager Architecture"
$Shape1.Cells('VerticalAlign') = 0
$Shape1.Cells('Para.HorzAlign') = 0
$Shape1.Cells('Char.Size').Formula = '20 pt'
$Shape1.Cells('Char.Color').Formula = '=RGB(255,255,255)'
$Line1 = $Page.DrawLine(0.3125, 10.3438, 12.4948, 10.3438)
$Line1.Cells('LineWeight').Formula = '1 pt'
$Line1.Cells('LineColor').Formula = '=RGB(255,255,255)'
# Step 4.
# Draw Client Rectangle, Set Size, Set Colour
# Set Text, Align
# Draw Line
$Shape2 = $Page.Drop($Rectangle, 1.7656, 9.2344)
$Shape2.Cells('Width').Formula = '2.7813'
$Shape2.Cells('Height').Formula = '1.9688'
$Shape2.Cells('FillForegnd').Formula = '=RGB(209,235,241)'
$Shape2.Cells('LinePattern').Formula = 1
$Shape2.Text = "Client"
$Shape2.Cells('VerticalAlign') = 0
$Shape2.Cells('Char.Size').Formula = '14 pt'
$Line2 = $Page.DrawLine(0.4297, 9.9427, 3.0833, 9.9427)
$Line2.Cells('LineWeight').Formula = '0.5 pt'
$Line2.Cells('LineColor').Formula = '=RGB(0,0,0)'
# Step 5.
# Add Computer Items Visio Stensils
# Set Masters item PC
$Stensil2 = $Application.Documents.Add("COMPS_M.vss")
$PC1 = $Stensil2.Masters.Item("PC")
# Step 6.
# Draw item PC
# Set Text, Size
$Shape3 = $Page.Drop($PC1, 1.1173, 9.1693)
$Shape3.Text = "Administrator Console"
$Shape3.Cells('Char.Size').Formula = '10 pt'
# Step 7.
# Draw Powershell Icon
# Set Position, Size
# Set Text
$Picture1 = $Page.Import("c:\!\powershell.png")
$Picture1.Cells('Width').Formula = '0.9843'
$Picture1.Cells('Height').Formula = '0.9843'
$Picture1.Cells('PinX').Formula = '2.5547'
$Picture1.Cells('PinY').Formula = '9.2682'
$Picture1.Text = "Windows Powershell"
$Picture1.Cells('Char.Size').Formula = '10 pt'
The Draw-VisioItem function has three mandatory parameters: Master – The name of the shape sample, and the X and Y coordinates of the location. The remaining parameters, such as: FillForegnd – the color of the shape, LinePattern – the line style of the shape outline, Text – The text of the shape, VerticalAlign and ParaHorzAlign – vertical and horizontal text alignment parameters, CharSize and CharColor – the size and color of the inscription characters are optional.
Function Draw-VisioItem ($Master, $X, $Y, $Width, $Height, $FillForegnd, $LinePattern, $Text, $VerticalAlign, $ParaHorzAlign, $CharSize, $CharColor) {
#######################################################################
#
# Powershell function for Draw Visio Item
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 15.09.2007
# Purpose/Change: Initial script development
#
# Version: 0.2
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 16.09.2007
# Purpose/Change: Add Flow Control Input Parameters
#
# Version: 0.3
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 17.09.2007
# Purpose/Change: Reorganize Variables
#
# Run:
#
# Draw-VisioItem -Master "Rectangle" -X 6.375 -Y 7.125 -Width 12.2501 -Height 7.25 -FillForegnd "RGB(0,153,204)"`
# -LinePattern 0 -Text "Microsoft Virtual Machine Manager Architecture" -VerticalAlign 0 -ParaHorzAlign 0`
# -CharSize "20 pt" -CharColor "RGB(255,255,255)"
#
#######################################################################
$Script:Shape++
$Master = $Master -replace " ",""
# Set Expression And Draw Item
$Expression = '$Script:Shape' + $Script:Shape + ' = $Script:Page.Drop(' + '$' + $Master + ',' + $X + ',' + $Y + ')'
Invoke-Expression $Expression
# Set Item Width Properties
If ($Width)
{
$Expression = '$Script:Shape' + $Script:Shape + '.Cells("Width").Formula = ' + $Width
Invoke-Expression $Expression
}
# Set Item Height Properties
If ($Height)
{
$Expression = '$Script:Shape' + $Script:Shape + '.Cells("Height").Formula = ' + $Height
Invoke-Expression $Expression
}
# Set Item FillForegnd Properties
If ($FillForegnd)
{
$Expression = '$Script:Shape' + $Script:Shape + '.Cells("FillForegnd").Formula = "=' + $FillForegnd + '"'
Invoke-Expression $Expression
}
# Set Item LinePattern Properties
If ($LinePattern)
{
$Expression = '$Script:Shape' + $Script:Shape + '.Cells("LinePattern").Formula = ' + $LinePattern
Invoke-Expression $Expression
}
# Set Item Text
If ($Text)
{
$Expression = '$Script:Shape' + $Script:Shape + '.Text = "' + $Text + '"'
Invoke-Expression $Expression
}
# Set Item VerticalAlign Properties
If ($VerticalAlign)
{
$Expression = '$Script:Shape' + $Script:Shape + '.Cells("VerticalAlign").Formula = ' + $VerticalAlign
Invoke-Expression $Expression
}
# Set Item HorzAlign Properties
If ($ParaHorzAlign)
{
$Expression = '$Script:Shape' + $Script:Shape + '.Cells("Para.HorzAlign").Formula = ' + $ParaHorzAlign
Invoke-Expression $Expression
}
# Set Item Char.Size Properties
If ($CharSize)
{
$Expression = '$Script:Shape' + $Script:Shape + '.Cells("Char.Size").Formula = "' + $CharSize + '"'
Invoke-Expression $Expression
}
# Set Item Char.Color Properties
If ($CharColor)
{
$Expression = '$Script:Shape' + $Script:Shape + '.Cells("Char.Color").Formula = "=' + $CharColor + '"'
Invoke-Expression $Expression
}
}
Draw-VisioLine
The Draw-VisioLine function is designed to draw a variety of lines. It has several mandatory parameters: coordinates of the beginning BeginX, BeginY, and the end coordinates EndX, EndY. Optional parameters can also be specified: LineColor – line color and arrow style BeginArrow, EndArrow.
Function Draw-VisioLine ($BeginX, $BeginY, $EndX, $EndY, $LineWeight, $LineColor, $BeginArrow, $EndArrow) {
#######################################################################
#
# Powershell function for Draw Visio Line
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 15.09.2007
# Purpose/Change: Initial script development
#
# Version: 0.2
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 17.09.2007
# Purpose/Change: Add Flow Control Input Parameters
#
# Run:
#
# Draw-VisioLine -BeginX 0.3125 -BeginY 10.3438 -EndX 12.4948 -EndY 10.3438 -LineWeight "1 pt"`
# -LineColor "RGB(255,255,255)" -BeginArrow 4 -EndArrow 4
#
#######################################################################
$Script:Line++
# Set Expression And Draw Line
$Expression = '$Script:Line' + $Script:Line + ' = $Script:Page.DrawLine(' + $BeginX + ',' + $BeginY + ',' + $EndX + ',' + $EndY + ')'
Invoke-Expression $Expression
# Set Line Width Properties
If ($LineWeight)
{
$Expression = '$Script:Line' + $Script:Line + '.Cells("LineWeight").Formula = "' + $LineWeight + '"'
Invoke-Expression $Expression
}
# Set Line Color Properties
$Expression = '$Script:Line' + $Script:Line + '.Cells("LineColor").Formula = "=' + $LineColor + '"'
Invoke-Expression $Expression
# Set Line Begin Arrow Properties
If ($BeginArrow)
{
$Expression = '$Script:Line' + $Script:Line + '.Cells("BeginArrow").Formula = ' + $BeginArrow
Invoke-Expression $Expression
}
# Set Line End Arrow Properties
If ($EndArrow)
{
$Expression = '$Script:Line' + $Script:Line + '.Cells("EndArrow").Formula = ' + $EndArrow
Invoke-Expression $Expression
}
}
Draw-VisioIcon
The Draw-VisioIcon function will allow you to add pre-prepared icons in .png or .jpeg format to schemes. It has several mandatory parameters: IconPath – path and file name of the icon, dimensions Width, Height and coordinates PinX, PinY. Optional parameters are the text of the label Text and its character size CharSize.
Function Draw-VisioIcon ($IconPath, $Width, $Height, $PinX, $PinY, $Text, $CharSize) {
#######################################################################
#
# Powershell function for Draw Visio Icon
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 16.09.2007
# Purpose/Change: Initial script development
#
# Version: 0.2
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 17.09.2007
# Purpose/Change: Add Flow Control Input Parameters
#
# Run:
#
# Draw-VisioIcon -IconPath "c:\!\powershell.png" -Width 0.9843 -Height 0.9843 -PinX 2.5547 -PinY 9.2682`
# -Text "Windows Powershell" -CharSize "10 pt"
#
#######################################################################
$Script:Icon++
# Import Icon Item
$Expression = '$Script:Icon' + $Script:Icon + ' = $Script:Page.Import("' + $IconPath + '")'
Invoke-Expression $Expression
# Set Icon Width Properties
$Expression = '$Script:Icon' + $Script:Icon + '.Cells("Width").Formula = ' + $Width
Invoke-Expression $Expression
# Set Icon Height Properties
$Expression = '$Script:Icon' + $Script:Icon + '.Cells("Height").Formula = ' + $Height
Invoke-Expression $Expression
# Set Icon PinX Properties
$Expression = '$Script:Icon' + $Script:Icon + '.Cells("PinX").Formula = ' + $PinX
Invoke-Expression $Expression
# Set Icon PinY Properties
$Expression = '$Script:Icon' + $Script:Icon + '.Cells("PinY").Formula = ' + $PinY
Invoke-Expression $Expression
# Set Icon Text
If ($Text)
{
$Expression = '$Script:Icon' + $Script:Icon + '.Text = "' + $Text + '"'
Invoke-Expression $Expression
}
# Set Icon Char.Size Properties
If ($CharSize)
{
$Expression = '$Script:Icon' + $Script:Icon + '.Cells("Char.Size").Formula = "' + $CharSize + '"'
Invoke-Expression $Expression
}
}
Resize-VisioPageToFitContents
The Resize-VisioPageToFitContents function is extremely simple, its task is to resize the page to fit the image. It has no input parameters.
Function Resize-VisioPageToFitContents {
#######################################################################
#
# Powershell function for Resize Active Visio Document Page to Fit Contents
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 17.09.2007
# Purpose/Change: Initial script development
#
# Run:
#
# Resize-VisioPageToFitContents
#
#######################################################################
# Resize Page to Fit Contents
$Script:Page.ResizeToFitContents()
}
Save-VisioDocument
Small final steps remain. The logic of the Save-VisioDocument function is to save the created diagram as a Visio .vsd file. The function has a mandatory File parameter – the name of the Visio .vsd file.
Function Save-VisioDocument ($File) {
#######################################################################
#
# Powershell function for save Visio Document
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 17.09.2007
# Purpose/Change: Initial script development
#
# Run:
#
# Save-VisioDocument -File 'C:\!\MsSCVMM2007Arch.vsd'
#
#######################################################################
# Save Document
$Expression = '$Script:Document.SaveAs("' + $File + '")'
Invoke-Expression $Expression
}
Close-VisioApplication
The Close-VisioApplication function completes this storyline. It has no parameters, and the logic is only in closing the Visio application.
Function Close-VisioApplication {
#######################################################################
#
# Powershell function for create Visio Application
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 17.09.2007
# Purpose/Change: Initial script development
#
# Run:
#
# Close-VisioApplication
#
#######################################################################
# Close Visio Application
$Script:Application.Quit()
}
Brief Summary
The final output of the function code in the PSVisio.v0.1.ps1 file looks like this:
#######################################################################
#
# Powershell functions for operate Visio Drawing
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 14.09.2007
# Purpose/Change: Initial script development.
# Create function New-VisioApplication.
#
# Version: 0.2
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Change Date: 14.09.2007
# Purpose/Change: Create function New-VisioDocument.
#
# Version: 0.3
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Change Date: 14.09.2007
# Purpose/Change: Create function Set-VisioPage.
#
# Version: 0.4
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Change Date: 15.09.2007
# Purpose/Change: Create function Add-VisioStensil.
#
# Version: 0.5
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Change Date: 15.09.2007
# Purpose/Change: Create function Set-VisioStensilMasterItem.
#
# Version: 0.6
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Change Date: 15.09.2007
# Purpose/Change: Create function Draw-VisioItem.
#
# Version: 0.7
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Change Date: 15.09.2007
# Purpose/Change: Create function Draw-VisioLine.
#
# Version: 0.8
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 16.09.2007
# Purpose/Change: Change function Draw-VisioItem.
#
# Version: 0.9
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Change Date: 16.09.2007
# Purpose/Change: Create function Draw-VisioIcon.
#
# Version: 0.10
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Change Date: 17.09.2007
# Purpose/Change: Change function Draw-VisioIcon.
#
# Version: 0.11
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Change Date: 17.09.2007
# Purpose/Change: Change function Draw-VisioLine.
#
# Version: 0.12
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Change Date: 17.09.2007
# Purpose/Change: Change function Set-VisioStensilMasterItem.
#
# Version: 0.13
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Change Date: 17.09.2007
# Purpose/Change: Change function Draw-VisioItem.
#
# Version: 0.14
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Change Date: 17.09.2007
# Purpose/Change: Add function Resize-VisioPageToFitContents.
#
# Version: 0.15
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Change Date: 17.09.2007
# Purpose/Change: Add function Save-VisioDocument.
#
#
# Version: 0.16
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Change Date: 18.09.2007
# Purpose/Change: Add function Draw-VisioText.
#
# Version: 0.17
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Change Date: 18.09.2007
# Purpose/Change: Add function Draw-VisioPolyLine.
#
# Version: 0.18
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Change Date: 19.09.2007
# Purpose/Change: Change function Draw-VisioText.
#
# Run:
#
# . .\PSVisio.v0.1.ps1
#
#######################################################################
# Set Variables
$Shape=0
$Line=0
$Icon=0
$Text=0
$PolyLine=0
Function New-VisioApplication {
#######################################################################
#
# Powershell function for create Visio Application
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 14.09.2007
# Purpose/Change: Initial script development
#
# Run:
#
# New-VisioApplication
#
#######################################################################
# Create Visio Object
$Script:Application = New-Object -ComObject Visio.Application
$Script:Application.Visible = $True
}
Function New-VisioDocument {
#######################################################################
#
# Powershell function for create Visio Document
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 14.09.2007
# Purpose/Change: Initial script development
#
# Run:
#
# New-VisioDocument
#
#######################################################################
# Create Document from Blank Template
$Script:Documents = $Script:Application.Documents
$Script:Document=$Script:Application.Documents.Add('')
}
Function Set-VisioPage {
#######################################################################
#
# Powershell function for Set Active Visio Document Page
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 14.09.2007
# Purpose/Change: Initial script development
#
# Run:
#
# Set-VisioPage
#
#######################################################################
# Set Visio Active Page
$Script:Page=$Script:Application.ActivePage
$Script:Application.ActivePage.PageSheet
}
Function Add-VisioStensil ($Name, $File) {
#######################################################################
#
# Powershell function for Add Visio Stensil
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 15.09.2007
# Purpose/Change: Initial script development
#
# Run:
#
# Add-VisioStensil -Name "Basic" -File "BASIC_M.vss"
#
#######################################################################
# Set Expression and Add Visio Stensil
$Expression = '$Script:' + $Name + ' = $Script:Application.Documents.Add("' + $File +'")'
Invoke-Expression $Expression
}
Function Set-VisioStensilMasterItem ($Stensil, $Item) {
#######################################################################
#
# Powershell function for Set Stensil Master Item
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 15.09.2007
# Purpose/Change: Initial script development
#
# Version: 0.2
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 17.09.2007
# Purpose/Change: Reorganize Variables
#
# Run:
#
# Set-VisioStensilMasterItem -Stensil "Basic" -Item "Rectangle"
#
#######################################################################
# Set Expression And Set Masters Item Rectangle
$ItemWithoutSpace = $Item -replace " ",""
$Expression = '$Script:' + $ItemWithoutSpace + ' = $Script:' + $Stensil + '.Masters.Item("' + $Item + '")'
Invoke-Expression $Expression
}
Function Draw-VisioItem ($Master, $X, $Y, $Width, $Height, $FillForegnd, $LinePattern, $Text, $VerticalAlign, $ParaHorzAlign, $CharSize, $CharColor) {
#######################################################################
#
# Powershell function for Draw Visio Item
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 15.09.2007
# Purpose/Change: Initial script development
#
# Version: 0.2
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 16.09.2007
# Purpose/Change: Add Flow Control Input Parameters
#
# Version: 0.3
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 17.09.2007
# Purpose/Change: Reorganize Variables
#
# Run:
#
# Draw-VisioItem -Master "Rectangle" -X 6.375 -Y 7.125 -Width 12.2501 -Height 7.25 -FillForegnd "RGB(0,153,204)"`
# -LinePattern 0 -Text "Microsoft Virtual Machine Manager Architecture" -VerticalAlign 0 -ParaHorzAlign 0`
# -CharSize "20 pt" -CharColor "RGB(255,255,255)"
#
#######################################################################
$Script:Shape++
$Master = $Master -replace " ",""
# Set Expression And Draw Item
$Expression = '$Script:Shape' + $Script:Shape + ' = $Script:Page.Drop(' + '$' + $Master + ',' + $X + ',' + $Y + ')'
Invoke-Expression $Expression
# Set Item Width Properties
If ($Width)
{
$Expression = '$Script:Shape' + $Script:Shape + '.Cells("Width").Formula = ' + $Width
Invoke-Expression $Expression
}
# Set Item Height Properties
If ($Height)
{
$Expression = '$Script:Shape' + $Script:Shape + '.Cells("Height").Formula = ' + $Height
Invoke-Expression $Expression
}
# Set Item FillForegnd Properties
If ($FillForegnd)
{
$Expression = '$Script:Shape' + $Script:Shape + '.Cells("FillForegnd").Formula = "=' + $FillForegnd + '"'
Invoke-Expression $Expression
}
# Set Item LinePattern Properties
If ($LinePattern)
{
$Expression = '$Script:Shape' + $Script:Shape + '.Cells("LinePattern").Formula = ' + $LinePattern
Invoke-Expression $Expression
}
# Set Item Text
If ($Text)
{
$Expression = '$Script:Shape' + $Script:Shape + '.Text = "' + $Text + '"'
Invoke-Expression $Expression
}
# Set Item VerticalAlign Properties
If ($VerticalAlign)
{
$Expression = '$Script:Shape' + $Script:Shape + '.Cells("VerticalAlign").Formula = ' + $VerticalAlign
Invoke-Expression $Expression
}
# Set Item HorzAlign Properties
If ($ParaHorzAlign)
{
$Expression = '$Script:Shape' + $Script:Shape + '.Cells("Para.HorzAlign").Formula = ' + $ParaHorzAlign
Invoke-Expression $Expression
}
# Set Item Char.Size Properties
If ($CharSize)
{
$Expression = '$Script:Shape' + $Script:Shape + '.Cells("Char.Size").Formula = "' + $CharSize + '"'
Invoke-Expression $Expression
}
# Set Item Char.Color Properties
If ($CharColor)
{
$Expression = '$Script:Shape' + $Script:Shape + '.Cells("Char.Color").Formula = "=' + $CharColor + '"'
Invoke-Expression $Expression
}
}
Function Draw-VisioLine ($BeginX, $BeginY, $EndX, $EndY, $LineWeight, $LineColor, $BeginArrow, $EndArrow) {
#######################################################################
#
# Powershell function for Draw Visio Line
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 15.09.2007
# Purpose/Change: Initial script development
#
# Version: 0.2
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 17.09.2007
# Purpose/Change: Add Flow Control Input Parameters
#
# Run:
#
# Draw-VisioLine -BeginX 0.3125 -BeginY 10.3438 -EndX 12.4948 -EndY 10.3438 -LineWeight "1 pt"`
# -LineColor "RGB(255,255,255)" -BeginArrow 4 -EndArrow 4
#
#######################################################################
$Script:Line++
# Set Expression And Draw Line
$Expression = '$Script:Line' + $Script:Line + ' = $Script:Page.DrawLine(' + $BeginX + ',' + $BeginY + ',' + $EndX + ',' + $EndY + ')'
Invoke-Expression $Expression
# Set Line Width Properties
If ($LineWeight)
{
$Expression = '$Script:Line' + $Script:Line + '.Cells("LineWeight").Formula = "' + $LineWeight + '"'
Invoke-Expression $Expression
}
# Set Line Color Properties
$Expression = '$Script:Line' + $Script:Line + '.Cells("LineColor").Formula = "=' + $LineColor + '"'
Invoke-Expression $Expression
# Set Line Begin Arrow Properties
If ($BeginArrow)
{
$Expression = '$Script:Line' + $Script:Line + '.Cells("BeginArrow").Formula = ' + $BeginArrow
Invoke-Expression $Expression
}
# Set Line End Arrow Properties
If ($EndArrow)
{
$Expression = '$Script:Line' + $Script:Line + '.Cells("EndArrow").Formula = ' + $EndArrow
Invoke-Expression $Expression
}
}
Function Draw-VisioIcon ($IconPath, $Width, $Height, $PinX, $PinY, $Text, $CharSize) {
#######################################################################
#
# Powershell function for Draw Visio Icon
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 16.09.2007
# Purpose/Change: Initial script development
#
# Version: 0.2
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 17.09.2007
# Purpose/Change: Add Flow Control Input Parameters
#
# Run:
#
# Draw-VisioIcon -IconPath "c:\!\powershell.png" -Width 0.9843 -Height 0.9843 -PinX 2.5547 -PinY 9.2682`
# -Text "Windows Powershell" -CharSize "10 pt"
#
#######################################################################
$Script:Icon++
# Import Icon Item
$Expression = '$Script:Icon' + $Script:Icon + ' = $Script:Page.Import("' + $IconPath + '")'
Invoke-Expression $Expression
# Set Icon Width Properties
$Expression = '$Script:Icon' + $Script:Icon + '.Cells("Width").Formula = ' + $Width
Invoke-Expression $Expression
# Set Icon Height Properties
$Expression = '$Script:Icon' + $Script:Icon + '.Cells("Height").Formula = ' + $Height
Invoke-Expression $Expression
# Set Icon PinX Properties
$Expression = '$Script:Icon' + $Script:Icon + '.Cells("PinX").Formula = ' + $PinX
Invoke-Expression $Expression
# Set Icon PinY Properties
$Expression = '$Script:Icon' + $Script:Icon + '.Cells("PinY").Formula = ' + $PinY
Invoke-Expression $Expression
# Set Icon Text
If ($Text)
{
$Expression = '$Script:Icon' + $Script:Icon + '.Text = "' + $Text + '"'
Invoke-Expression $Expression
}
# Set Icon Char.Size Properties
If ($CharSize)
{
$Expression = '$Script:Icon' + $Script:Icon + '.Cells("Char.Size").Formula = "' + $CharSize + '"'
Invoke-Expression $Expression
}
}
Function Resize-VisioPageToFitContents {
#######################################################################
#
# Powershell function for Resize Active Visio Document Page to Fit Contents
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 17.09.2007
# Purpose/Change: Initial script development
#
# Run:
#
# Resize-VisioPageToFitContents
#
#######################################################################
# Resize Page to Fit Contents
$Script:Page.ResizeToFitContents()
}
Function Save-VisioDocument ($File) {
#######################################################################
#
# Powershell function for save Visio Document
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 17.09.2007
# Purpose/Change: Initial script development
#
# Run:
#
# Save-VisioDocument -File 'C:\!\MsSCVMM2007Arch.vsd'
#
#######################################################################
# Save Document
$Expression = '$Script:Document.SaveAs("' + $File + '")'
Invoke-Expression $Expression
}
Function Close-VisioApplication {
#######################################################################
#
# Powershell function for create Visio Application
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 17.09.2007
# Purpose/Change: Initial script development
#
# Run:
#
# Close-VisioApplication
#
#######################################################################
# Close Visio Application
$Script:Application.Quit()
}
Function Draw-VisioText ($X, $Y, $Width, $Height, $FillForegnd, $LinePattern, $Text, $VerticalAlign, $ParaHorzAlign, $CharSize, $CharColor, $CharStyle, $FillForegndTrans) {
#######################################################################
#
# Powershell function for Draw Visio Text Label
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 18.09.2007
# Purpose/Change: Initial script development
#
# Version: 0.2
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 19.09.2007
# Purpose/Change: Add CharStyle Parameter
#
# Run:
#
# Draw-VisioText -X 4.25 -Y 8.875 -Width 1.3751 -Height 0.375 -Text "Microsoft Virtual Machine Manager Architecture"`
# -LinePattern "0" -FillForegndTrans "100%" -CharStyle 17
#
#######################################################################
$Script:Text++
$Master = "Rectangle"
# Set Expression And Draw Text
$Expression = '$Script:Text' + $Script:Text + ' = $Script:Page.Drop(' + '$' + $Master + ',' + $X + ',' + $Y + ')'
Invoke-Expression $Expression
# Set Item Width Properties
If ($Width)
{
$Expression = '$Script:Text' + $Script:Text + '.Cells("Width").Formula = ' + $Width
Invoke-Expression $Expression
}
# Set Item Height Properties
If ($Height)
{
$Expression = '$Script:Text' + $Script:Text + '.Cells("Height").Formula = ' + $Height
Invoke-Expression $Expression
}
# Set Item FillForegnd Properties
If ($FillForegnd)
{
$Expression = '$Script:Text' + $Script:Text + '.Cells("FillForegnd").Formula = "=' + $FillForegnd + '"'
Invoke-Expression $Expression
}
# Set Item LinePattern Properties
If ($LinePattern)
{
$Expression = '$Script:Text' + $Script:Text + '.Cells("LinePattern").Formula = ' + $LinePattern
Invoke-Expression $Expression
}
# Set Item Text
If ($Text)
{
$Expression = '$Script:Text' + $Script:Text + '.Text = "' + $Text + '"'
Invoke-Expression $Expression
}
# Set Item VerticalAlign Properties
If ($VerticalAlign)
{
$Expression = '$Script:Text' + $Script:Text + '.Cells("VerticalAlign").Formula = ' + $VerticalAlign
Invoke-Expression $Expression
}
# Set Item HorzAlign Properties
If ($ParaHorzAlign)
{
$Expression = '$Script:Text' + $Script:Text + '.Cells("Para.HorzAlign").Formula = ' + $ParaHorzAlign
Invoke-Expression $Expression
}
# Set Item Char.Size Properties
If ($CharSize)
{
$Expression = '$Script:Text' + $Script:Text + '.Cells("Char.Size").Formula = "' + $CharSize + '"'
Invoke-Expression $Expression
}
# Set Item Char.Color Properties
If ($CharColor)
{
$Expression = '$Script:Text' + $Script:Text + '.Cells("Char.Color").Formula = "=' + $CharColor + '"'
Invoke-Expression $Expression
}
# Set Item Char.Style Properties
If ($CharStyle)
{
$Expression = '$Script:Text' + $Script:Text + '.Cells("Char.Style").Formula = "' + $CharStyle + '"'
Invoke-Expression $Expression
}
# Set Item FillForegndTrans Properties
If ($FillForegndTrans)
{
$Expression = '$Script:Text' + $Script:Text + '.Cells("FillForegndTrans").Formula = "' + $FillForegndTrans + '"'
Invoke-Expression $Expression
}
}
Function Draw-VisioPolyLine ($PolyLine, $LineWeight, $LineColor, $BeginArrow, $EndArrow) {
#######################################################################
#
# Powershell function for Draw Visio PolyLine
#
# Version: 0.1
# Author: Andrii Romanenko
# Website: blogs.airra.net
# Creation Date: 18.09.2007
# Purpose/Change: Initial script development
#
# Run:
#
# Draw-VisioPolyLine -Polyline 1.0938,9.0625,1.4063,9.0625,1.4063,8.6563,1.0938,8.6563 -LineWeight "0.5 pt"`
# -LineColor "RGB(255,255,255)" -BeginArrow 1 -EndArrow 1
#
#######################################################################
$Script:PolyLine++
[double[]]$PolyLineCoordinates=@()
$PolyLineCoordinates += $Polyline
# Set Expression And Draw PolyLine
$Expression = '$Script:PolyLine' + $Script:PolyLine + ' = $Script:Page.DrawPolyLine(($PolyLineCoordinates),0)'
Invoke-Expression $Expression
# Set Line Width Properties
If ($LineWeight)
{
$Expression = '$Script:PolyLine' + $Script:PolyLine + '.Cells("LineWeight").Formula = "' + $LineWeight + '"'
Invoke-Expression $Expression
}
# Set Line Color Properties
$Expression = '$Script:PolyLine' + $Script:PolyLine + '.Cells("LineColor").Formula = "=' + $LineColor + '"'
Invoke-Expression $Expression
# Set Line Begin Arrow Properties
If ($BeginArrow)
{
$Expression = '$Script:PolyLine' + $Script:PolyLine + '.Cells("BeginArrow").Formula = ' + $BeginArrow
Invoke-Expression $Expression
}
# Set Line End Arrow Properties
If ($EndArrow)
{
$Expression = '$Script:PolyLine' + $Script:PolyLine + '.Cells("EndArrow").Formula = ' + $EndArrow
Invoke-Expression $Expression
}
}
Accordingly, the code for forming the Microsoft System Center Virtual Machine Manager 2007 system architecture diagram in Visio format:
. .\PSVisio.v0.1.ps1
# Step 1.
# Create Visio Application
# Create Document from Blank Template
# Set Active Page
New-VisioApplication
New-VisioDocument
Set-VisioPage
# Step 2.
# Add Basic Visio Stensils
# Set Masters Item Rectangle
Add-VisioStensil -Name "Basic" -File "BASIC_M.vss"
Set-VisioStensilMasterItem -Stensil Basic -Item "Rectangle"
# Step 3.
# Draw Main Rectangle, Set Size, Set Colour
# Set Text, Size, Color, Align
# Draw Line, Set Weight, Color
Draw-VisioItem -Master "Rectangle" -X 6.375 -Y 7.125 -Width 12.2501 -Height 7.25 -FillForegnd "RGB(0,153,204)"`
-LinePattern 0 -Text "Microsoft Virtual Machine Manager Architecture" -VerticalAlign '0' -ParaHorzAlign '0'`
-CharSize "20 pt" -CharColor "RGB(255,255,255)"
Draw-VisioLine -BeginX 0.3125 -BeginY 10.3438 -EndX 12.4948 -EndY 10.3438 -LineWeight "1 pt" -LineColor "RGB(255,255,255)"
# Step 4.
# Draw Client Rectangle, Set Size, Set Colour
# Set Text, Align
# Draw Line
Draw-VisioItem -Master "Rectangle" -X 1.7656 -Y 9.2344 -Width 2.7813 -Height 1.9688 -FillForegnd "RGB(209,235,241)"`
-LinePattern 1 -Text "Client" -VerticalAlign '0' -ParaHorzAlign '1'`
-CharSize "14 pt" -CharColor "RGB(0,0,0)"
Draw-VisioLine -BeginX 0.4297 -BeginY 9.9427 -EndX 3.0833 -EndY 9.9427 -LineWeight "0.5 pt" -LineColor "RGB(0,0,0)"
# Step 5.
# Add Computer Items Visio Stensils
# Set Masters item PC
Add-VisioStensil -Name "Computer" -File "COMPS_M.vss"
Set-VisioStensilMasterItem -Stensil "Computer" -Item "PC"
# Step 6.
# Draw item PC
# Set Text, Size
Draw-VisioItem -Master "PC" -X 1.1173 -Y 9.1693 -Text "Administrator Console" -CharSize "10 pt"
# Step 7.
# Draw Powershell Icon
# Set Position, Size
# Set Text
Draw-VisioIcon -IconPath "c:\!\powershell.png" -Width 0.9843 -Height 0.9843 -PinX 2.5547 -PinY 9.2682 `
-Text "Windows Powershell" -CharSize "10 pt"
# Step 8.
# Draw WCF Rectangle, Set Size, Set Colour
# Set Text, Align
# Draw Line
Draw-VisioItem -Master "Rectangle" -X 4.2682 -Y 6.9063 -Width 1.5 -Height 6.625 -FillForegnd "RGB(255,255,255)"`
-LinePattern 1 -Text "Windows Communication Foundation" -VerticalAlign '0'`
-CharSize "14 pt"
Draw-VisioLine -BeginX 3.5664 -BeginY 9.4063 -EndX 4.9492 -EndY 9.4063 -LineWeight "0.5 pt" -LineColor "RGB(0,0,0)"
# Step 9.----
# Draw WCF Icon
# Set Position, Size
Draw-VisioIcon -IconPath "c:\!\WCF.png" -Width 1.412 -Height 1.0443 -PinX 4.2708 -PinY 6.9779
# Step 10.
# Draw Line communication from Client to WCF
# Set Arrow
Draw-VisioLine -BeginX 3.1563 -BeginY 9.2396 -EndX 3.5174 -EndY 9.2396 -LineColor "RGB(255,255,255)" -BeginArrow 4 -EndArrow 4
# Step 11.
# Draw Web Client Rectangle, Set Size, Set Colour
# Set Text, Align
# Draw Line
Draw-VisioItem -Master "Rectangle" -X 1.7656 -Y 6.8776 -Width 2.7813 -Height 2.1094 -FillForegnd "RGB(209,235,241)"`
-LinePattern 1 -Text "Web Client" -VerticalAlign '0'`
-CharSize "14 pt"
Draw-VisioLine -BeginX 0.4297 -BeginY 7.6562 -EndX 3.0833 -EndY 7.6562 -LineWeight "0.5 pt" -LineColor "RGB(0,0,0)"
# Step 12.
# Draw Self Service Portal Icon
# Set Position, Size
# Set Text
Draw-VisioIcon -IconPath "c:\!\SelfServicePortal.png" -Width 0.8438 -Height 0.8438 -PinX 1.1094 -PinY 6.9271 `
-Text "Self Service Portal" -CharSize "10 pt"
# Step 13.
# Draw Powershell Icon
# Set Position, Size
# Set Text
Draw-VisioIcon -IconPath "c:\!\powershell.png" -Width 0.9843 -Height 0.9843 -PinX 2.4922 -PinY 6.9192 `
-Text "Windows Powershell" -CharSize "10 pt"
# Step 14.
# Draw Line communication from Web Client to WCF
# Set Arrow
Draw-VisioLine -BeginX 3.158 -BeginY 6.8802 -EndX 3.5191 -EndY 6.8802 -LineColor "RGB(255,255,255)" -BeginArrow 4 -EndArrow 4
# Step 15.
# Draw Scripting Client Rectangle, Set Size, Set Colour
# Set Text, Align
# Draw Line
Draw-VisioItem -Master "Rectangle" -X 1.7657 -Y 4.5469 -Width 2.7813 -Height 1.9062 -FillForegnd "RGB(209,235,241)"`
-LinePattern 1 -Text "Scripting Client" -VerticalAlign '0'`
-CharSize "14 pt"
Draw-VisioLine -BeginX 0.4297 -BeginY 5.1979 -EndX 3.0834 -EndY 5.1979 -LineWeight "0.5 pt" -LineColor "RGB(0,0,0)"
# Step 16.
# Draw Powershell Icon
# Set Position, Size
# Set Text
Draw-VisioIcon -IconPath "c:\!\powershell.png" -Width 0.9843 -Height 0.9843 -PinX 1.7631 -PinY 4.6015 `
-Text "Windows Powershell" -CharSize "10 pt"
# Step 17.
# Draw Line communication from Scripting Client to WCF
# Set Arrow
Draw-VisioLine -BeginX 3.158 -BeginY 4.5729 -EndX 3.5191 -EndY 4.5729 -LineColor "RGB(255,255,255)" -BeginArrow 4 -EndArrow 4
# Step 18.
# Draw Microsoft System Center
# Virtual Machine Manager Server 2007 Rectangle
# Set Size, Set Colour
# Set Text, Align
Draw-VisioItem -Master "Rectangle" -X 6.3281 -Y 8.2812 -Width 1.5 -Height 2.8125 -FillForegnd "RGB(255,192,0)"`
-LinePattern 1 -Text "Microsoft System Center Virtual Machine Manager Server 2007" -CharSize "14 pt"
# Step 19.
# Draw Microsoft SQL Server 2005 Rectangle
# Set Size, Set Colour
# Set Text, Align
Draw-VisioItem -Master "Rectangle" -X 6.3281 -Y 4.9219 -Width 1.5 -Height 2.6563 -FillForegnd "RGB(255,192,0)"`
-LinePattern 1 -Text "Microsoft SQL Server 2005" -CharSize "14 pt"
# Step 20.
# Add Server Items Visio Stensils
# Set Masters item Management Server and Database Server
Add-VisioStensil -Name "Server" -File "SERVER_M.vss"
Set-VisioStensilMasterItem -Stensil "Server" -Item "Management server"
Set-VisioStensilMasterItem -Stensil "Server" -Item "Database server"
# Step 21.
# Draw item Management Server
Draw-VisioItem -Master "Management server" -X 5.6043 -Y 9.7421
# Step 22.
# Draw item Management Server
Draw-VisioItem -Master "Database server" -X 5.5832 -Y 6.3046
# Step 23.
# Draw Line communication from WCF to Microsoft System Center
# Virtual Machine Manager Server 2007
# Set Arrow
Draw-VisioLine -BeginX 5.0052 -BeginY 8.2604 -EndX 5.6024 -EndY 8.2604 -LineColor "RGB(255,255,255)" -BeginArrow 4 -EndArrow 4
# Step 24.
# Draw Line communication from Microsoft System Center
# Virtual Machine Manager Server 2007 to Microsoft SQL Server 2005
# Set Arrow
Draw-VisioLine -BeginX 6.3272 -BeginY 6.2344 -EndX 6.3272 -EndY 6.8802 -LineColor "RGB(255,255,255)" -BeginArrow 4 -EndArrow 0
# Step 25.
# Draw Win-RM Rectangle, Set Size, Set Colour
# Set Text, Align
# Draw Line
Draw-VisioItem -Master "Rectangle" -X 8.4375 -Y 6.9219 -Width 1.5 -Height 6.6563 -FillForegnd "RGB(255,255,255)"`
-LinePattern 1 -Text "Windows Remote Managemet (Win-RM)" -VerticalAlign '0'`
-CharSize "14 pt"
Draw-VisioLine -BeginX 7.75 -BeginY 9.1875 -EndX 9.1328 -EndY 9.1875 -LineWeight "0.5 pt" -LineColor "RGB(0,0,0)"
# Step 26.
# Draw Win-RM Icon
# Set Position, Size
Draw-VisioIcon -IconPath "c:\!\Win-RM.png" -Width 1.1563 -Height 1.1563 -PinX 8.4293 -PinY 7
# Step 27.
# Draw Line communication from Win-RM to Microsoft System Center
# Virtual Machine Manager Server 2007
# Set Arrow
Draw-VisioLine -BeginX 7.0781 -BeginY 8.2812 -EndX 7.6753 -EndY 8.2812 -LineColor "RGB(255,255,255)" -BeginArrow 4 -EndArrow 4
# Step 28.
# Draw P2V Source Rectangle, Set Size, Set Colour
# Set Text, Align
# Draw Line
Draw-VisioItem -Master "Rectangle" -X 10.9844 -Y 9.5 -Width 2.7813 -Height 1.5 -FillForegnd "RGB(209,235,241)"`
-LinePattern 1 -Text "P2V Source" -VerticalAlign '0'`
-CharSize "14 pt"
Draw-VisioLine -BeginX 9.6485 -BeginY 9.9739 -EndX 12.3021 -EndY 9.9739 -LineWeight "0.5 pt" -LineColor "RGB(0,0,0)"
# Step 29.
# Draw VMM Agent Icon
# Set Position, Size
# Set Text
Draw-VisioIcon -IconPath "c:\!\VMMAgent.png" -Width 0.8438 -Height 0.8438 -PinX 10.2969 -PinY 9.5 `
-Text "VMM Agent" -CharSize "10 pt"
# Step 30.
# Draw Powershell Icon
# Set Position, Size
# Set Text
Draw-VisioIcon -IconPath "c:\!\powershell.png" -Width 0.7968 -Height 0.7968 -PinX 11.6016 -PinY 9.6016 `
-Text "Windows Powershell" -CharSize "10 pt"
# Step 31.
# Draw Line communication from P2V Source to Win-RM
# Set Arrow
Draw-VisioLine -BeginX 9.1875 -BeginY 9.487 -EndX 9.599 -EndY 9.487 -LineColor "RGB(255,255,255)" -BeginArrow 4 -EndArrow 4
# Step 32.
# Draw Host Rectangle, Set Size, Set Colour
# Set Text, Align
# Draw Line
Draw-VisioItem -Master "Rectangle" -X 10.9792 -Y 6.9427 -Width 2.7813 -Height 1.5 -FillForegnd "RGB(209,235,241)"`
-LinePattern 1 -Text "Host" -VerticalAlign '0'`
-CharSize "14 pt"
Draw-VisioLine -BeginX 9.6472 -BeginY 7.4219 -EndX 12.3008 -EndY 7.4219 -LineWeight "0.5 pt" -LineColor "RGB(0,0,0)"
# Step 33.
# Draw VMM Agent Icon
# Set Position, Size
# Set Text
Draw-VisioIcon -IconPath "c:\!\VMMAgent.png" -Width 0.8438 -Height 0.8438 -PinX 10.2917 -PinY 6.9427 `
-Text "VMM Agent" -CharSize "10 pt"
# Step 34.
# Draw Microsoft Virtual Server 2005 R2 Icon
# Set Position, Size
# Set Text
Draw-VisioIcon -IconPath "c:\!\WS.jpg" -Width 0.8125 -Height 0.7404 -PinX 11.5938 -PinY 6.9423 `
-Text "Microsoft Virtual Server 2005 R2" -CharSize "7 pt"
# Step 35.
# Draw Line communication from Host to Win-RM
# Set Arrow
Draw-VisioLine -BeginX 9.1849 -BeginY 6.941 -EndX 9.5964 -EndY 6.941 -LineColor "RGB(255,255,255)" -BeginArrow 4 -EndArrow 4
# Step 36.
# Draw Library Rectangle, Set Size, Set Colour
# Set Text, Align
# Draw Line
Draw-VisioItem -Master "Rectangle" -X 10.9792 -Y 4.3542 -Width 2.7813 -Height 1.5 -FillForegnd "RGB(209,235,241)"`
-LinePattern 1 -Text "Library" -VerticalAlign '0'`
-CharSize "14 pt"
Draw-VisioLine -BeginX 9.6419 -BeginY 4.8073 -EndX 12.2955 -EndY 4.8073 -LineWeight "0.5 pt" -LineColor "RGB(0,0,0)"
# Step 37.
# Draw VMM Agent Icon
# Set Position, Size
# Set Text
Draw-VisioIcon -IconPath "c:\!\VMMAgent.png" -Width 0.8438 -Height 0.8438 -PinX 10.2917 -PinY 4.3542 `
-Text "VMM Agent" -CharSize "10 pt"
# Step 38.
# Draw Windows Server 2003 R2 Icon
# Set Position, Size
# Set Text
Draw-VisioIcon -IconPath "c:\!\WS.jpg" -Width 0.8125 -Height 0.7404 -PinX 11.5625 -PinY 4.3702 `
-Text "Windows Server 2003 R2" -CharSize "7 pt"
# Step 39.
# Draw Line communication from Library to Win-RM
# Set Arrow
Draw-VisioLine -BeginX 9.1901 -BeginY 4.3611 -EndX 9.6016 -EndY 4.3611 -LineColor "RGB(255,255,255)" -BeginArrow 4 -EndArrow 4
# Step 40.
# Draw BITS Rectangle, Set Size, Set Colour
# Set Text, Align
# Draw Line
Draw-VisioItem -Master "Rectangle" -X 10.9844 -Y 8.2292 -Width 2.75 -Height 0.5625 -FillForegnd "RGB(255,255,0)"`
-LinePattern 1 -Text "BITS" -ParaHorzAlign '0'`
-CharSize "14 pt"
# Step 41.
# Draw BITS Icon
# Set Position, Size
# Set Text
Draw-VisioIcon -IconPath "c:\!\BITS.jpg" -Width 0.5417 -Height 0.5417 -PinX 10.974 -PinY 8.2344
# Step 42.
# Draw Line communication from BITS to P2V Source
# Set Arrow
Draw-VisioLine -BeginX 10.9844 -BeginY 8.5104 -EndX 10.9844 -EndY 8.75 -LineColor "RGB(255,255,255)" -BeginArrow 4 -EndArrow 4
# Step 43.
# Draw Line communication from BITS to Host
# Set Arrow
Draw-VisioLine -BeginX 10.9844 -BeginY 7.6849 -EndX 10.9844 -EndY 7.9219 -LineColor "RGB(255,255,255)" -BeginArrow 4 -EndArrow 4
# Step 44.
# Draw BITS Rectangle, Set Size, Set Colour
# Set Text, Align
# Draw Line
Draw-VisioItem -Master "Rectangle" -X 10.9844 -Y 5.6555 -Width 2.75 -Height 0.5625 -FillForegnd "RGB(255,255,0)"`
-LinePattern 1 -Text "BITS" -ParaHorzAlign '0'`
-CharSize "14 pt"
# Step 45.
# Draw BITS Icon
# Set Position, Size
# Set Text
Draw-VisioIcon -IconPath "c:\!\BITS.jpg" -Width 0.5417 -Height 0.5417 -PinX 10.974 -PinY 5.6607
# Step 46.
# Draw Line communication from BITS to Host
# Set Arrow
Draw-VisioLine -BeginX 10.9844 -BeginY 5.9245 -EndX 10.9844 -EndY 6.1901 -LineColor "RGB(255,255,255)" -BeginArrow 4 -EndArrow 4
# Step 47.
# Draw Line communication from BITS to Library
# Set Arrow
Draw-VisioLine -BeginX 10.9792 -BeginY 5.1042 -EndX 10.9792 -EndY 5.375 -LineColor "RGB(255,255,255)" -BeginArrow 4 -EndArrow 4
# Step 48.
# Resise Page To Fit Contents
Resize-VisioPageToFitContents
# Step 49.
# Save Document
# And Quit Application
Save-VisioDocument -File 'C:\!\MsSCVMM2007Arch.vsd'
Close-VisioApplication
Therefore, the final result is completely similar to the one described in previous publications, but optimization using functions allowed to reduce the volume of the code from 516 lines to 326. The code became more optimal and readable. The code reuse strategy is also a positive factor.
However, there are still tasks to be implemented: the functions should have a code for checking the input parameters for correctness, and add and expand algorithms for working with more complex shapes.
But that will be discussed in the next publications.
See you.
Sincerely, AIRRA!